- 该类可以解析开闭区间的数据,如图所示:
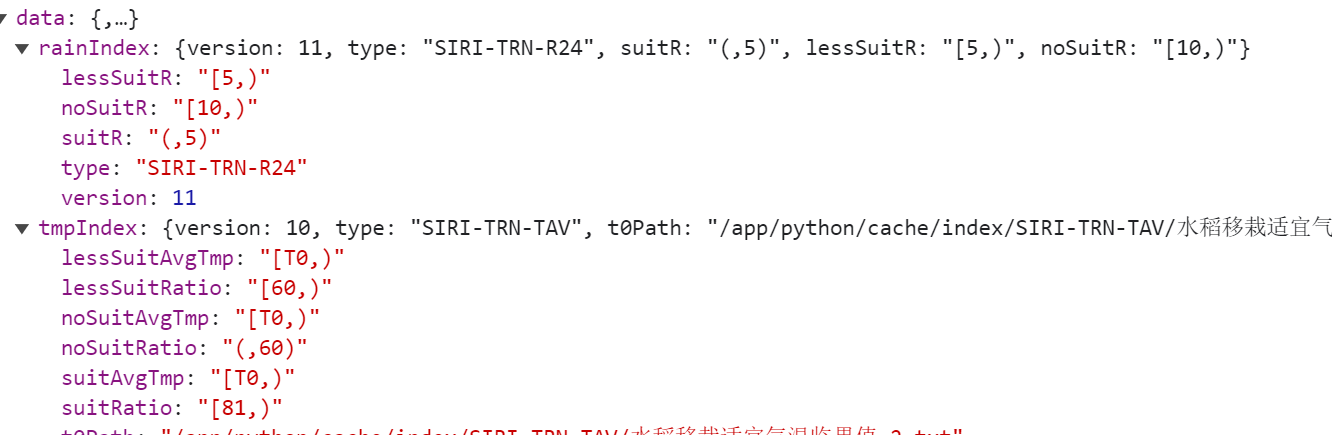
/**
* 解析某个数据 比如 suitTmp: '(0, 30]'
*/
export class IndexAnalyse {
/**
* 阈值(保留最新的括号字符串)
*/
thresholdValue: string;
/**
* 左数字
*/
private _leftValue: number;
public get leftValue(): number {
return this._leftValue;
}
public set leftValue(value: number) {
this._leftValue = value;
// 修改左数字的时候更新str的值
this.thresholdValue = this.getThresholdValue();
}
/**
* 右数字
*/
private _rightValue: number;
public get rightValue(): number {
return this._rightValue;
}
public set rightValue(value: number) {
this._rightValue = value;
// 修改右数字的时候更新str的值
this.thresholdValue = this.getThresholdValue();
}
/**
* 左符号
*/
private _leftSymbol: string;
public get leftSymbol(): string {
return this._leftSymbol;
}
/**
* 右符号
*/
private _rightSymbol: string;
public get rightSymbol(): string {
return this._rightSymbol;
}
/**
* 构造函数
*
* @param thresholdValue 阈值
*/
constructor(thresholdValue: string) { // thresholdValue: [1,2) [1,) [,2)
// 保留着括号形式的字符串
if (thresholdValue === '') {
this.thresholdValue = '';
return;
}
this.thresholdValue = thresholdValue;
// 解析左边括号
let leftBrackets = '';
let rightBrackets = '';
if (/\(/.test(thresholdValue)) {
leftBrackets = '(';
this._leftSymbol = '<';
} else {
leftBrackets = '[';
this._leftSymbol = '≤';
}
// 解析右边括号
if (/\)/.test(thresholdValue)) {
rightBrackets = ')';
this._rightSymbol = '<';
} else {
rightBrackets = ']';
this._rightSymbol = '≤';
}
// 去掉左右括号 例:1,2
thresholdValue = thresholdValue.replace(leftBrackets, '');
thresholdValue = thresholdValue.replace(rightBrackets, '');
// 分割之后得到左右数
this.leftValue = thresholdValue.split(',')[0] ? +thresholdValue.split(',')[0] : undefined;
this.rightValue = thresholdValue.split(',')[1] ? +thresholdValue.split(',')[1] : undefined;
// 左边没数字->左边符号应为''
if (this.leftValue === undefined) {
this._leftSymbol = '';
}
// 右边没数字->右边符号应为''
if (this.rightValue === undefined) {
this._rightSymbol = '';
}
}
/**
* 获取阈值(获取括号形态的字符串) 例:[0,2]
*
* @returns
*/
getThresholdValue(): string {
let leftBrackets = '';
let rightBrackets = '';
// 解析左符号
if (this._leftSymbol === '<' || this._leftSymbol === '') {
leftBrackets = '(';
} else if (this._leftSymbol === '≤') {
leftBrackets = '[';
}
// 解析右符号
if (this._rightSymbol === '<' || this._rightSymbol === '') {
rightBrackets = ')';
} else if (this._rightSymbol === '≤') {
rightBrackets = ']';
}
return `${leftBrackets}${this.leftValue !== undefined ? this.leftValue : ''},${this.rightValue !== undefined ? this.rightValue : ''}${rightBrackets}`;
}
/**
* 获取符号形态的字符串 例:0≤ d ≤2
*
* @param label
* @returns
*/
getRange(label: string): string {
if (this.leftValue && this.rightValue) {
return `${this.leftValue}${this._leftSymbol}${label}${this._rightSymbol}${this.rightValue}`;
} if (this.leftValue && !this.rightValue) {
return `${this.leftValue}${this._leftSymbol}${label}`;
} if (this.rightValue && !this.leftValue) {
return `${label}${this._rightSymbol}${this.rightValue}`;
}
return '';
}
}
// data 是如上图所示要解析的数据
const OBJ = data;
// 解析指标数据
for (const KEY in data) {
if (typeof data[KEY] !== 'number' && KEY !== 'type') {
OBJ[KEY] = new IndexAnalyse(data[KEY]);
}
}
console.log(OBJ);
- 返回的结果如下:
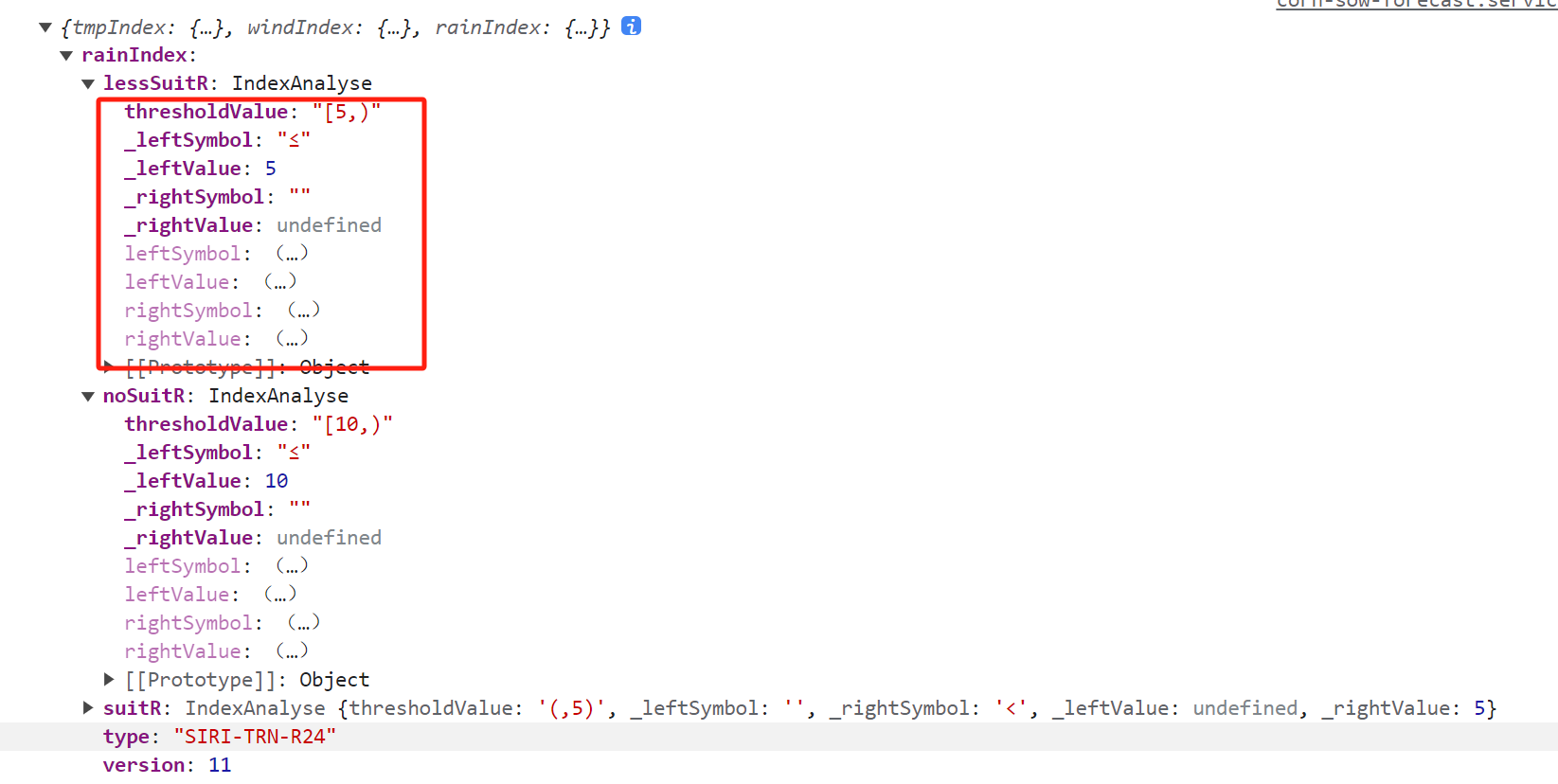
- html书写如下:
<tr>
<td class="active">适宜</td>
<td>降水量(mm)</td>
<td>
{{index.suitR.leftSymbol}}
{{index.suitR.rightSymbol}}
<span>
{{index.suitR.leftValue}}
{{index.suitR.rightValue}}
</span>
</td>
</tr>
- 页面展示如下:
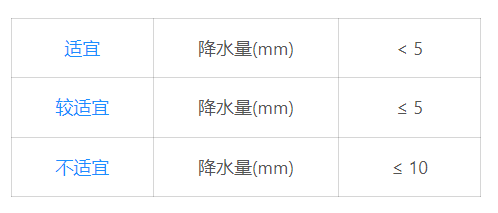